This article will guide us on how to interact with our Google Cloud Platform account using Python code. Our goal here is to get the instance’s data like its name, internal IP, and status.
Overview:
- Setup local python environment
- Install the Google API Python client module
- Setup and Create the JSON key file from GCP
- Interact with the GCP Compute Engine resource.
- Fetch the GCP instances details
Setup local python environment and activate.
The code below shows I created a virtual environment called gcp
and CD to that folder.
C:\Users\JMC\python>python -m venv gcp
C:\Users\JMC\python>cd gcp
C:\Users\JMC\python\gcp>dir
Volume in drive C has no label.
Volume Serial Number is AA01-5686
Directory of C:\Users\JMC\python\gcp
03/28/2022 07:08 PM <DIR> .
03/28/2022 07:08 PM <DIR> ..
03/28/2022 07:08 PM <DIR> Include
03/28/2022 07:08 PM <DIR> Lib
03/28/2022 07:08 PM 164 pyvenv.cfg
03/28/2022 07:08 PM <DIR> Scripts
1 File(s) 164 bytes
5 Dir(s) 455,774,732,288 bytes free
The code below where I activated the env then it shows gcp
on the terminal
C:\Users\JMC\python\gcp\Scripts>activate.bat
(gcp) C:\Users\JMC\python\gcp\Scripts>cd ..
Install the Google API Python client module
Create a file requirements.txt inside that env folder then run pip install -r requirements.txt
.
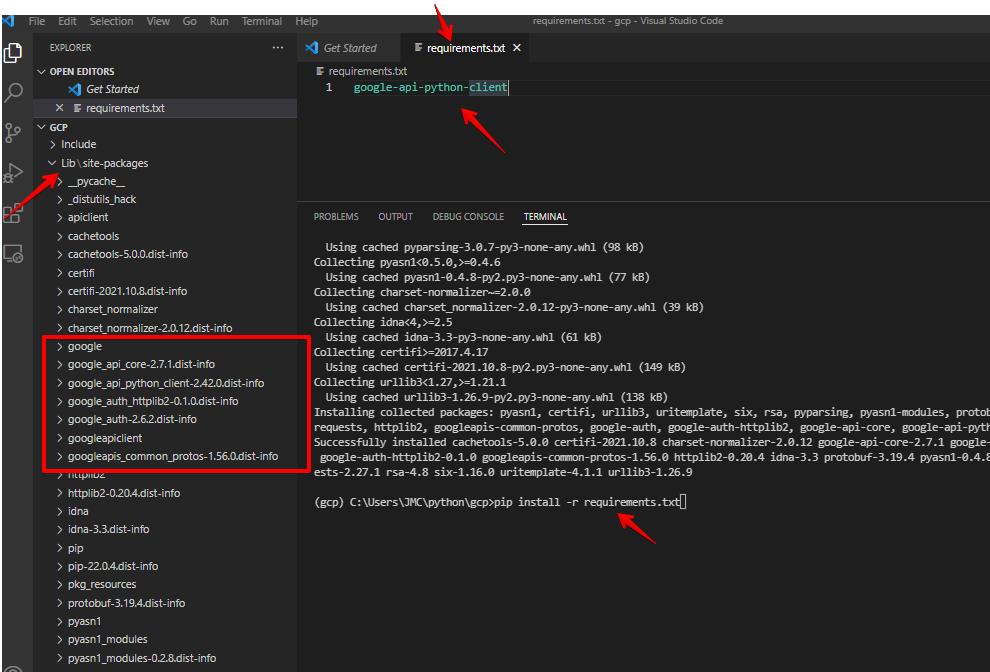
Import the GCP auth module and start creating a basic Python class and method
from google.oauth2 import service_account
class gcp():
def get_instances(self):
print('On this method `get_instances` will get start interacting with our GCP instances')
check = gcp();
check.get_instances()
(gcp) C:\Users\JMC\python\gcp>python gcp.py
On this method `get_instances` will get start interacting with our GCP instances
Setup and Create the JSON key file from GCP
- Follow this guide to create a GPC service account (GSA) and export the JSON key file for our Python authentication later.
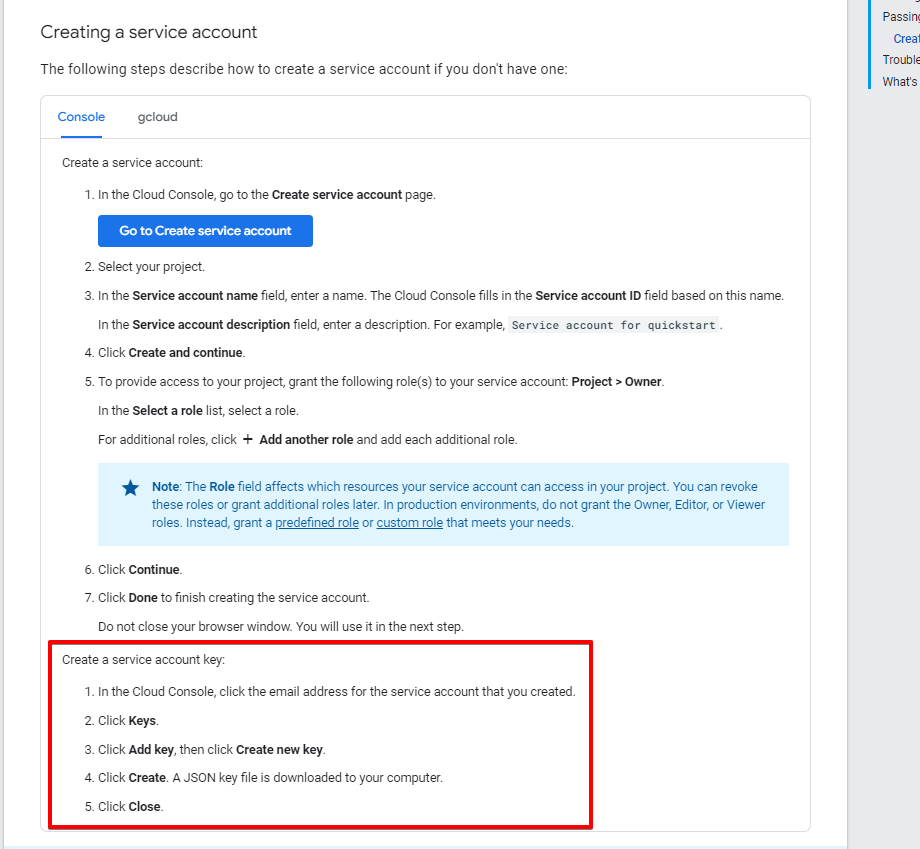
- Create a credential variable in Python to call that JSON file then import a module
googleapiclient.discovery
to interact with the GCP Compute Engine resource.
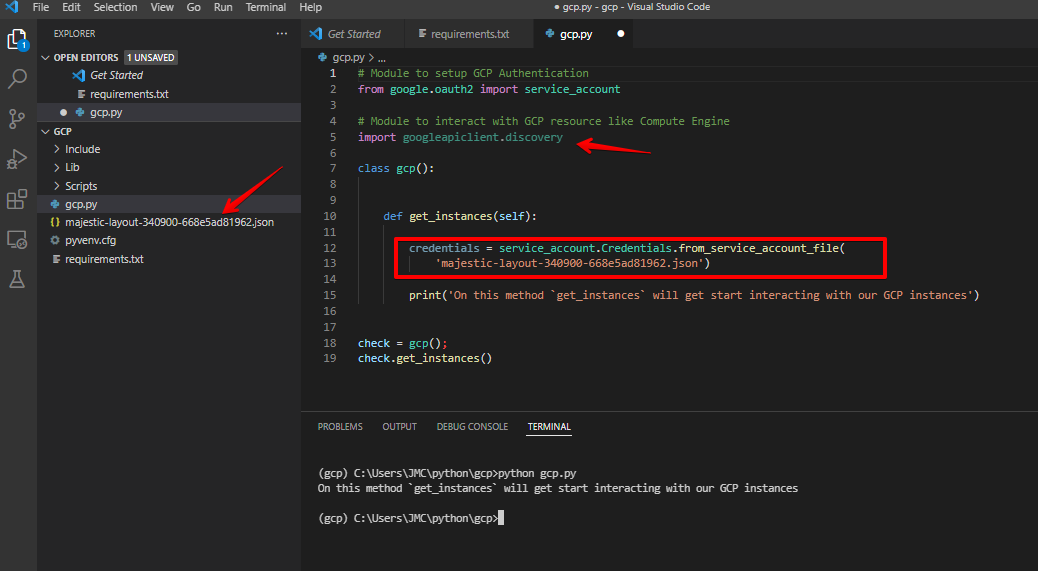
# Module to setup GCP Authentication
from google.oauth2 import service_account
# Module to interact with GCP resource like Compute Engine
import googleapiclient.discovery
class gcp():
def get_instances(self):
credentials = service_account.Credentials.from_service_account_file(
'majestic-layout-340900-668e5ad81962.json')
print('On this method `get_instances` will get start interacting with our GCP instances')
check = gcp();
check.get_instances()
Interact with the GCP Compute Engine resource.
- Setup a variable like
compute
.
compute = googleapiclient.discovery.build('compute', 'v1', credentials=credentials)
- Set up a variable
results
to list the instances.aggregatedList
is to fetch all instances in all regions and zones in GCP
result = compute.instances().aggregatedList(project=project_s).execute()
# Module to setup GCP Authentication
from google.oauth2 import service_account
# Module to interact with GCP resource like Compute Engine
import googleapiclient.discovery
class gcp():
def get_instances(self):
project = 'majestic-layout-340900'
credentials = service_account.Credentials.from_service_account_file(
'majestic-layout-340900-668e5ad81962.json')
print('On this method `get_instances` will get start interacting with our GCP instances')
# Setup variable to interact with GCP Compute engine
compute = googleapiclient.discovery.build('compute', 'v1', credentials=credentials)
# Setup a variable to list the intances
result = compute.instances().aggregatedList(project=project).execute()
print(result)
check = gcp();
check.get_instances()
Don’t also forget to add your project ID like set the project
variable
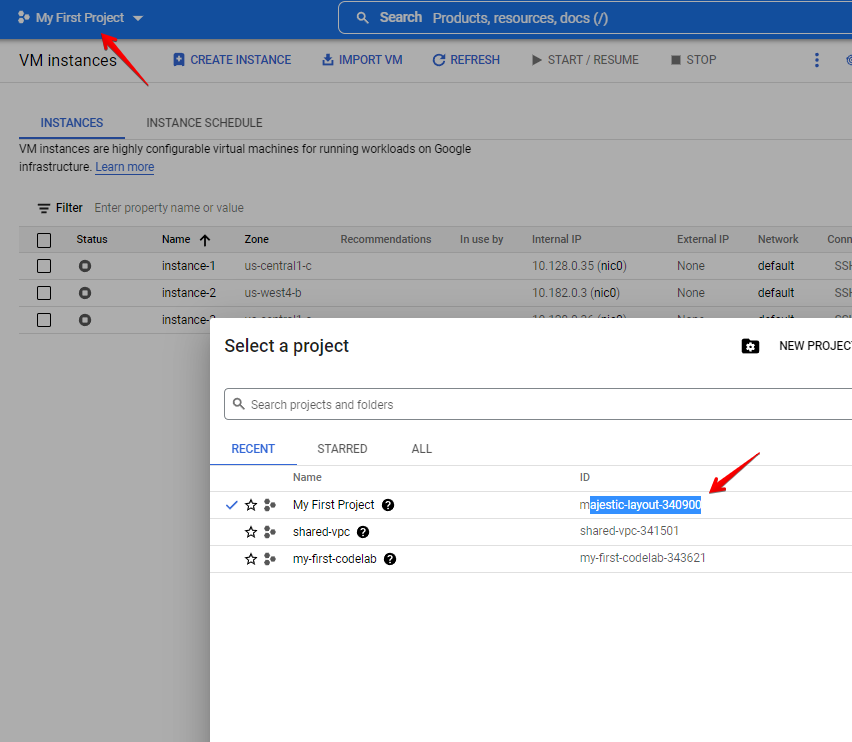
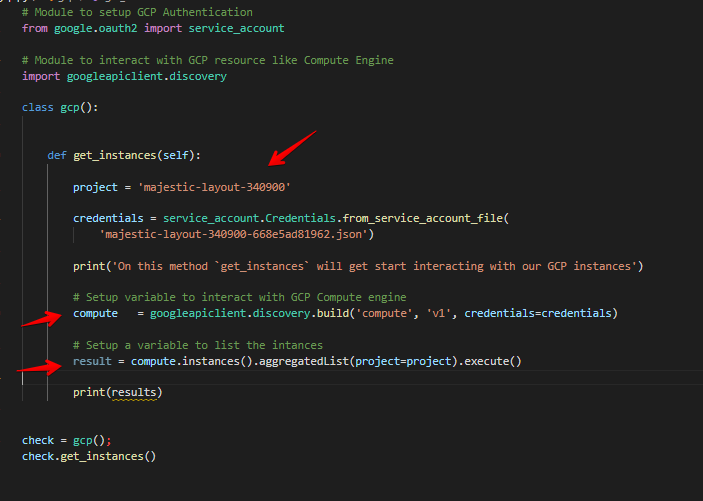
- Get the results! If you print the
result
variable, you will get a dictionary or some kind of associative array with a key value pair. Example: {“name”: “instance”} but you need to set up a loop to get what you need from that dictionary result like instance name, status, IP, etc.
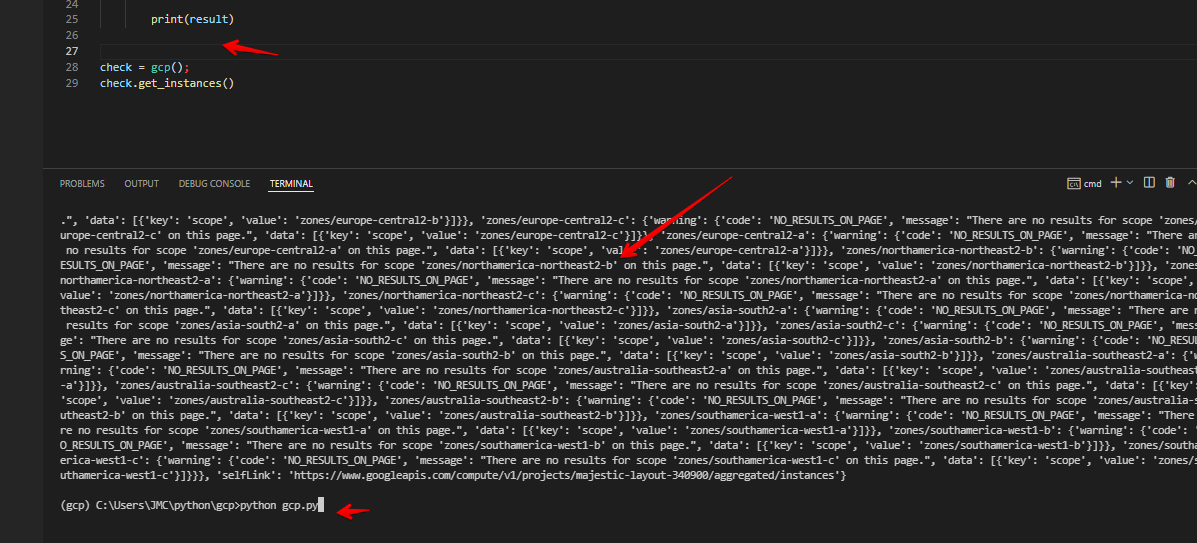
- Get the instance details like name, status, and ip.
If you will try to use a parser like https://json.parser.online.fr/
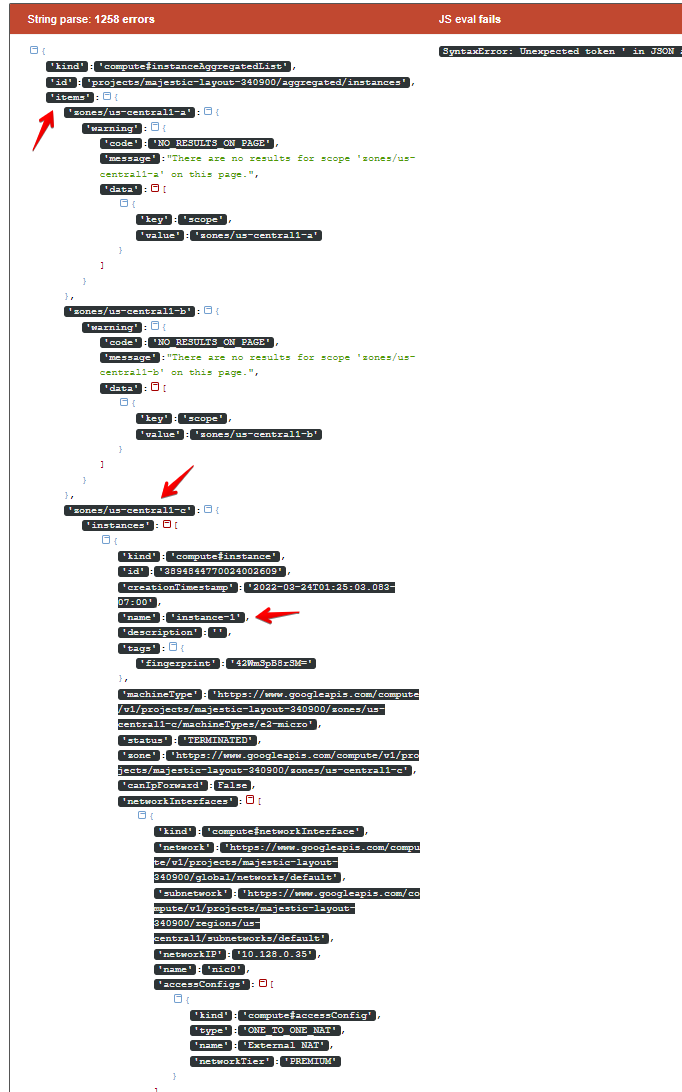
Fetch the GCP instances details
Now it is time to achieve our goal! Let’s the the Instances (virtual machines) name, status and IP.
- We need a loop to check and fetch the items from the
result
variable. So it goes something like this:- Fetch all data from
items
– The screenshot above there are 3 first-level keys:kind
,id
anditems
. The one we need is theitem
key like the screenshot above shows where the instances are located. - Look only for
instances
– Screenshot above shows we don’t have instances for other regions so that’s why we need this. - Fetch all data from
instances
then setup their variables like name, status, ip. - Print it!
- Fetch all data from
for _, labels_scoped_list in result['items'].items():
# Loop all values for the key 'instances' only
if 'instances' in labels_scoped_list:
for entry in labels_scoped_list['instances']:
# Print instance name, status and internal IP
instance_name = entry['name']
instance_status = entry['status']
instance_int_ip = entry['networkInterfaces'][0]['networkIP']
print(instance_name, instance_status, instance_int_ip)
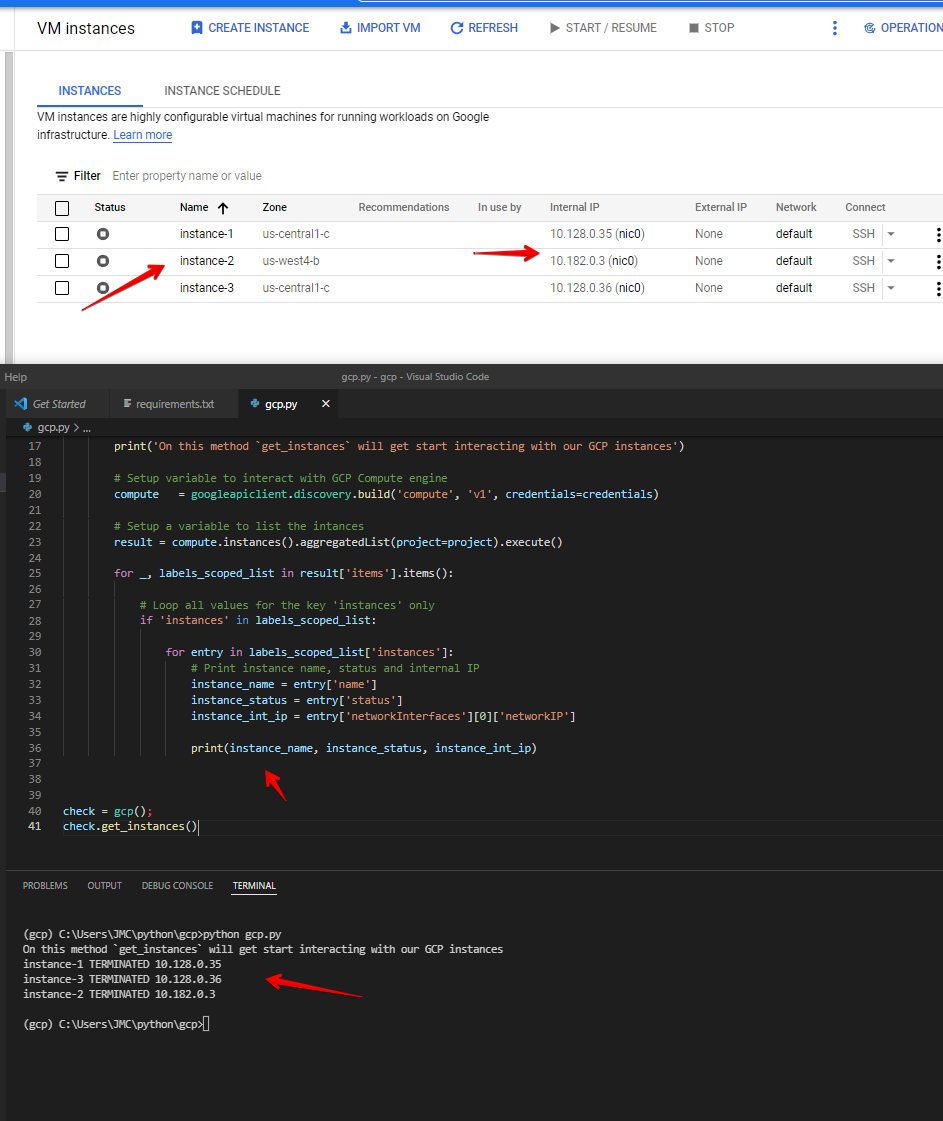
The screenshot above shows we’ve successfully created a Python script that interacts with our GCP account resource Compute Engine that fetches the instance’s details like name, status, and IP.