This article will guide us to the basic steps on how to wrap your codes (for example a basic HTML file) to a docker image, upload it to docker hub so we can use it later for Kubernetes deployment, and run a deployment in K8s using that image.
I will list some steps on how to:
- Build your codes as a docker image
- Upload it to Docker hub
- Deploy that docker in Kubernetes as a deployment (a simple nginx server)
Build your code
Our code is just a simple HTML file called test.html
and we are on the directory C:\Users\JMC\docker\simple-nginx

test.html
<html>
<body>
<title>This is a test nginx from a docker image deploying to K8s</title>
<h1>This is a test.html file. Welcome to the HTML world!</h1>
</body>
</html>
The docker file
The code below is the docker file where it tells docker to build and image with:
- an Ubuntu 20.04 base OS
- Maintainer
- Update and install Nginx
- Copy the test.html file to the default nginx public directory
- Install systemd and enable startup for nginx
# Base container OS image
FROM ubuntu:20.04
# Update the container repo
RUN apt-get update -y
# Install nginx and curl
RUN apt-get install nginx -y
RUN apt-get install curl -y
# Copy your file to the container
# This will copy the html file to the nginx public default DIR - /usr/share/nginx/www
COPY test.html /var/www/html/
# Expost Port 80
EXPOSE 80:80
# Run Nginx with daemon off
STOPSIGNAL SIGTERM
CMD ["nginx", "-g", "daemon off;"]
Build the image
Use this command to build a docker image from that dockerfiler
docker image build -t jmcausing1/simple-nginx:0.1 .
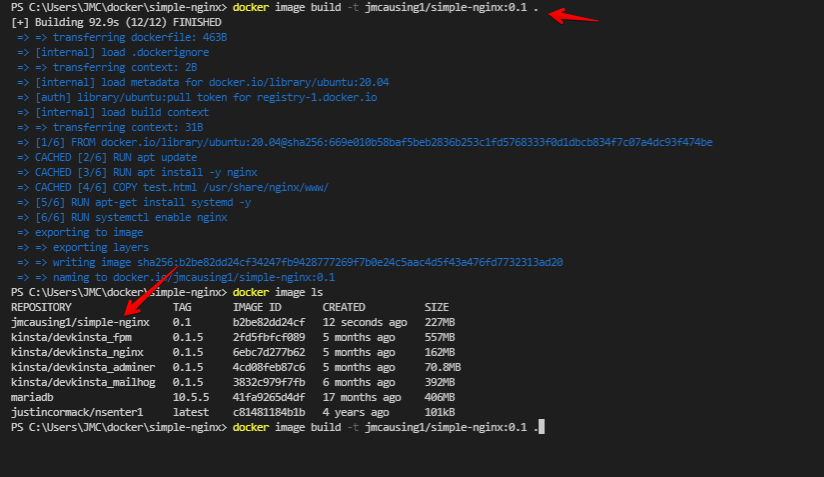
jmcausing1/simple-nginx
Upload it to Docker hub
Make sure you have a docker hub account. If not, register here https://hub.docker.com/
Then login to docker from your terminal/command prompt. Example:
docker login
Login with your Docker ID to push and pull images from Docker Hub. If you don't have a Docker ID, head over to https://hub.docker.com to create one.
Username: jmcausing1
Password:
Push/upload the image to your docker hub account
docker image push jmcausing1/simple-nginx:0.1
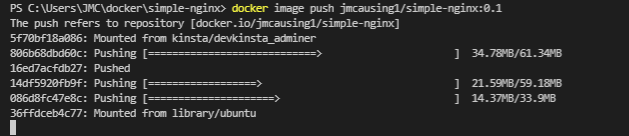
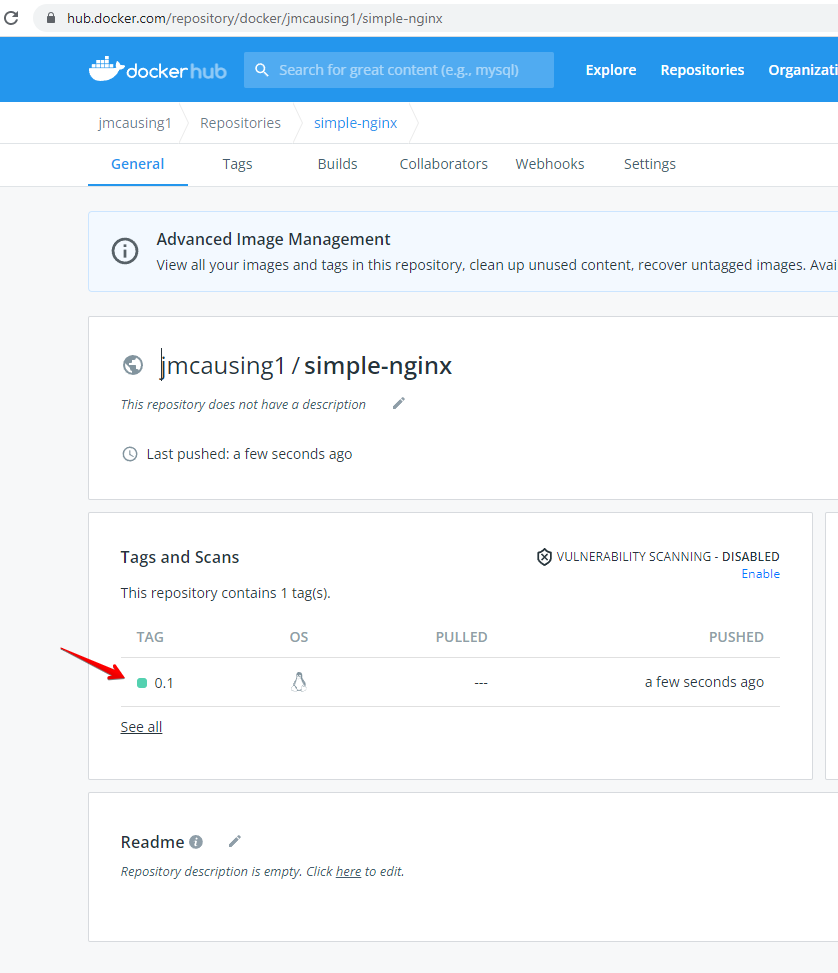
Deploy to Kubernetes
The yml file below will run a deployment with 3 replicas that will get an image container from docker hub called jmcausing1/simple-nginx:0.1
web-deploy.yml
apiVersion: apps/v1
kind: Deployment
metadata:
name: web
labels:
customer: acg
spec:
selector:
matchLabels:
app: web
replicas: 1
template:
metadata:
labels:
app: web
spec:
containers:
- image: jmcausing1/mynginx:1.0
name: web-ctr
Our Kubernetes cluster runs on Google Cloud (GKE)
kubectl get nodes
NAME STATUS ROLES AGE VERSION
gke-my-first-cluster-1-default-pool-afa2d638-7xtz Ready <none> 49s v1.22.4-gke.1501
gke-my-first-cluster-1-default-pool-afa2d638-gnvx Ready <none> 49s v1.22.4-gke.1501
gke-my-first-cluster-1-default-pool-afa2d638-xp0t Ready <none> 49s v1.22.4-gke.1501
Get the pod name
kubectl get pod -o wide
NAME READY STATUS RESTARTS AGE IP NODE NOMINATED NODE READINESS GATES
web-6845c95b74-dx4kr 1/1 Running 0 54s 10.104.2.37 gke-my-first-cluster-1-default-pool-afa2d638-7xtz <none> <none>
Describe the pod
kubectl describe pod web-6845c95b74-dx4kr
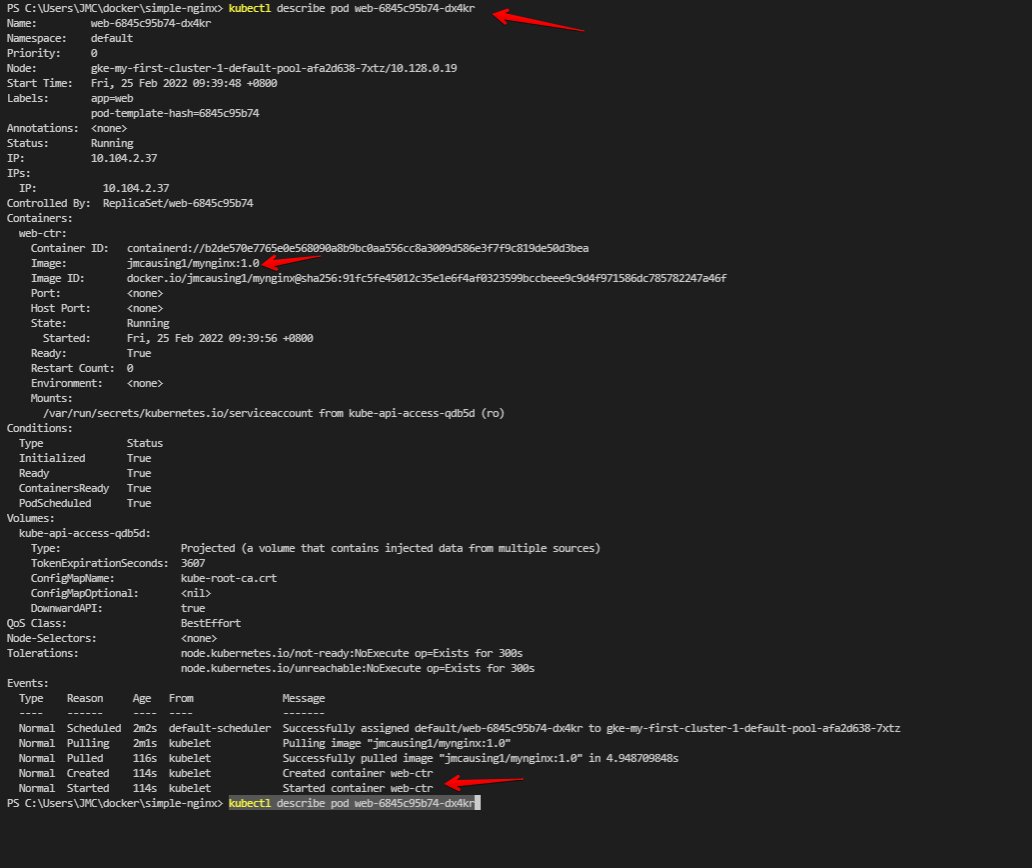
SSH to that container and test the nginx web server
kubectl exec web-6845c95b74-dx4kr -ti -- bash
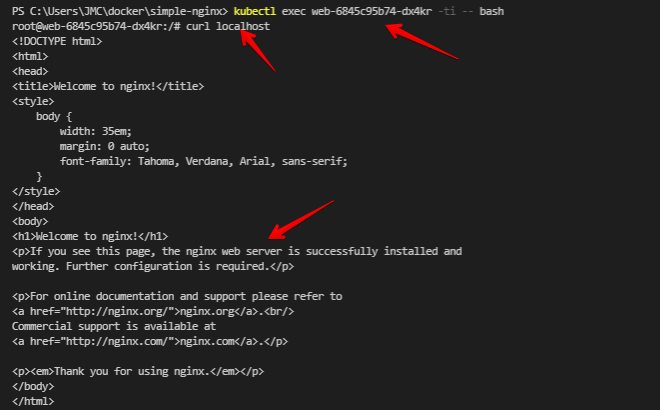
Expose the nginx web server
To expose the deployment that has the container with nginx web server, we need a kubernetes service.
web-lb.yml
apiVersion: v1
kind: Service
metadata:
name: web-svc
labels:
app: web
spec:
type: LoadBalancer
ports:
- port: 80
targetPort: 80
selector:
app: web
Apply the service yml file
kubectl apply -f web-lb.yml
Get the service details like its external IP
kubectl get svc -o wide
NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE SELECTOR
kubernetes ClusterIP 10.108.0.1 <none> 443/TCP 4d17h <none>
web-svc LoadBalancer 10.108.11.94 35.202.17.36 80:32066/TCP 6m3s app=web
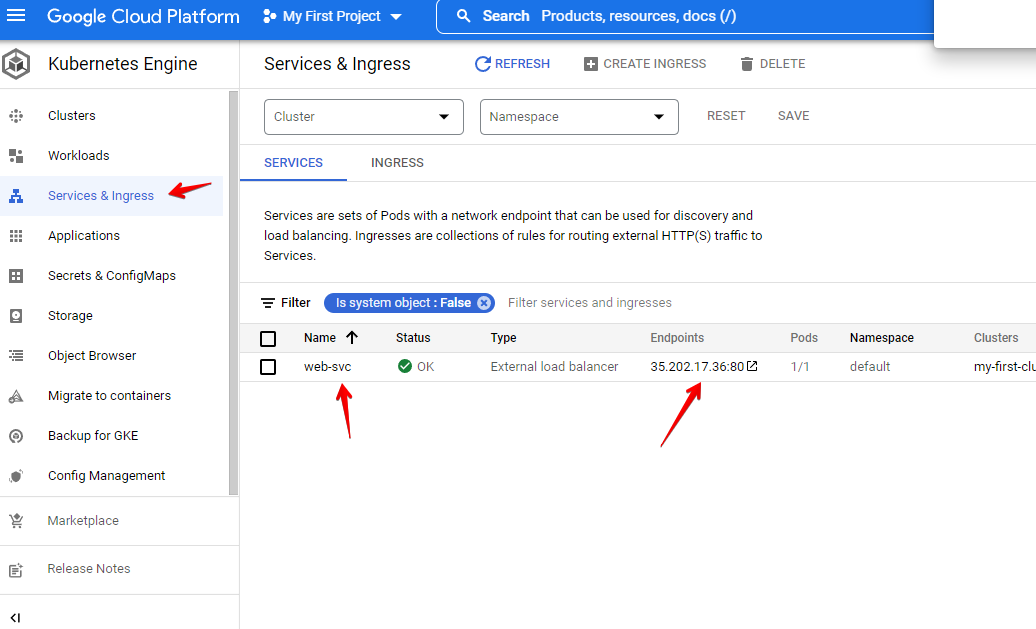
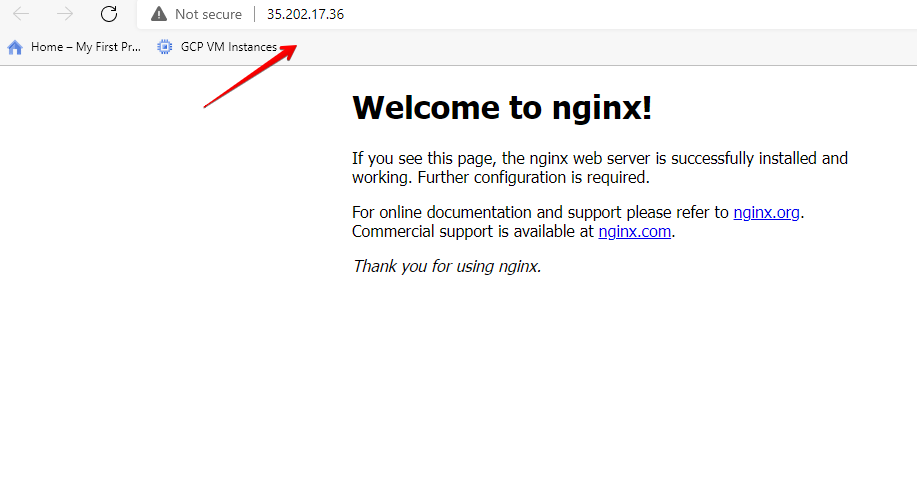
Remember our docker file we included these test HTML file?
# Copy your file to the container
# This will copy the html file to the nginx public default DIR - /usr/share/nginx/www
COPY test.html /var/www/html/
It’s there now!
